The Importance of Negative Testing
Automated tests often focus on the “happy path”—what happens when everything goes as expected. However, real-world scenarios require us to dig deeper and test how our applications handle unexpected or invalid actions. This is where negative testing comes in. Negative testing ensures that your application can handle invalid actions gracefully, providing robustness and reliability.
In this article, we’ll explore how to implement negative testing with Selenium, using practical examples from Instagram’s website. We’ll cover different scenarios, including character limit tests, missing required field tests, and missing UI component tests.
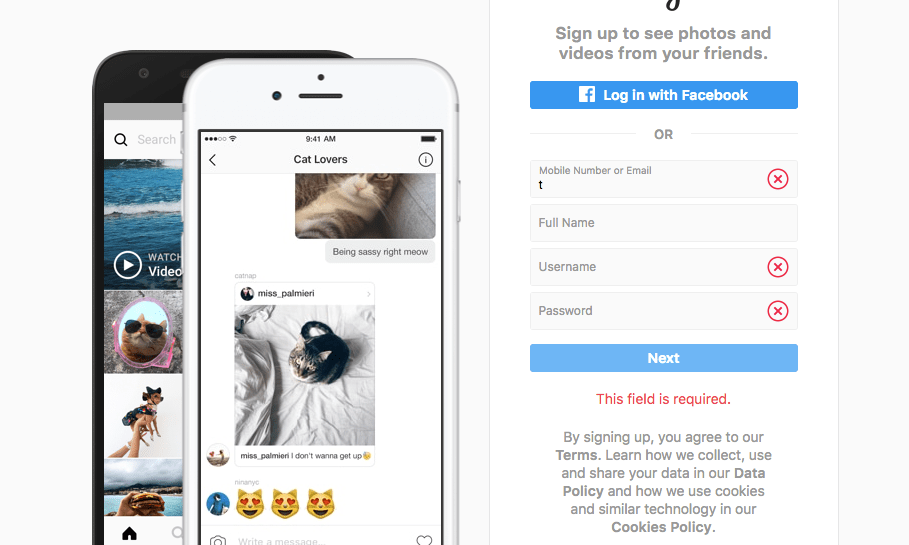
What is Negative Testing?
According to SmartBear, negative testing ensures that the application under test can handle invalid actions gracefully. In other words, negative tests check what happens when things go wrong. These tests are crucial for validating that your application can handle errors and edge cases effectively.
Character Limit Tests
Character limits are a common feature in web forms. They prevent users from entering more characters than allowed. Testing these limits ensures that the application behaves correctly when users exceed the character limit.
Example: Instagram’s Username Field
Instagram’s username field allows a maximum of 30 characters. To test this, we’ll:
- Locate the username field.
- Send more than 50 characters to it.
- Validate that the field contains no more than 30 characters.
Steps:
1. Locate the Element:
import pytest as pytest
from selenium.webdriver.common.by import By
from selenium.webdriver.support import expected_conditions as EC
from selenium.webdriver.support.wait import WebDriverWait
class SignUpPage:
def init(self, driver):
self.driver = driver
self.username_textbox = WebDriverWait(self.driver.instance, 5).until(
EC.visibility_of_element_located((By.NAME, "username")))
2. Send Characters:
def send_username(self, username):
self.username_textbox.send_keys(username)
3. Validate Character Limit:
def validate_username_length(self):
value = self.username_textbox.get_attribute("value")
assert len(value) <= 30
4. Run the Test:
python3 -m pytest testcases/test_instagram.py -k test_verify_username_character_limit
Missing Required Field Tests
Forms often have required fields that must be filled out before submission. Negative tests ensure that the application correctly identifies and handles missing required fields.
Example: Instagram’s Sign-Up Form
We’ll test a scenario where the user submits the form with missing required fields.
Steps:
1. Locate the Element:
class SignUpPage:
def init(self, driver):
self.mobile_email_textbox = WebDriverWait(self.driver.instance, 5).until(
EC.visibility_of_element_located((By.NAME, "emailOrPhone")))
2. Send Invalid Data:
def send_mobile_email(self, email):
self.mobile_email_textbox.send_keys(email)
3. Validate Errors:
def validate_required_field_errors(self):
errors = self.driver.find_elements(By.CLASS_NAME, "coreSpriteInputError gBp1f")
assert len(errors) == 3
4. Run the Test:
python3 -m pytest testcases/test_instagram.py -k test_verify_required_fields
Missing UI Component Tests
Sometimes, you need to ensure that a particular UI component is not present in the DOM. This can be tricky since Selenium cannot directly search for non-existent elements.
Example: Instagram Login Button
We’ll test that the login button is not present when the user is already logged in.
Steps:
1. Write Validation Method:
def validate_login_button_absence(self):
try:
self.driver.find_element(By.XPATH, "//button[text()='Login']")
assert False, "Login button should not be present"
except NoSuchElementException:
pass
2. Run the Test:
python3 -m pytest testcases/test_instagram.py -k test_login_button_is_not_present
Final Thoughts
Negative testing is essential for ensuring that your application can handle unexpected and invalid actions gracefully. By incorporating these tests into your Selenium automation suite, you can improve your application’s robustness and reliability. Start implementing negative testing today to catch potential issues before they affect your users.
For more in-depth tutorials and to access the source code, visit our GitHub repository.
Ready to Enhance Your Testing Strategy?
If you’re ready to take your testing strategy to the next level, dive deeper into negative testing with Selenium. Implement these techniques and ensure your web applications are robust and reliable. Don’t wait—start today!